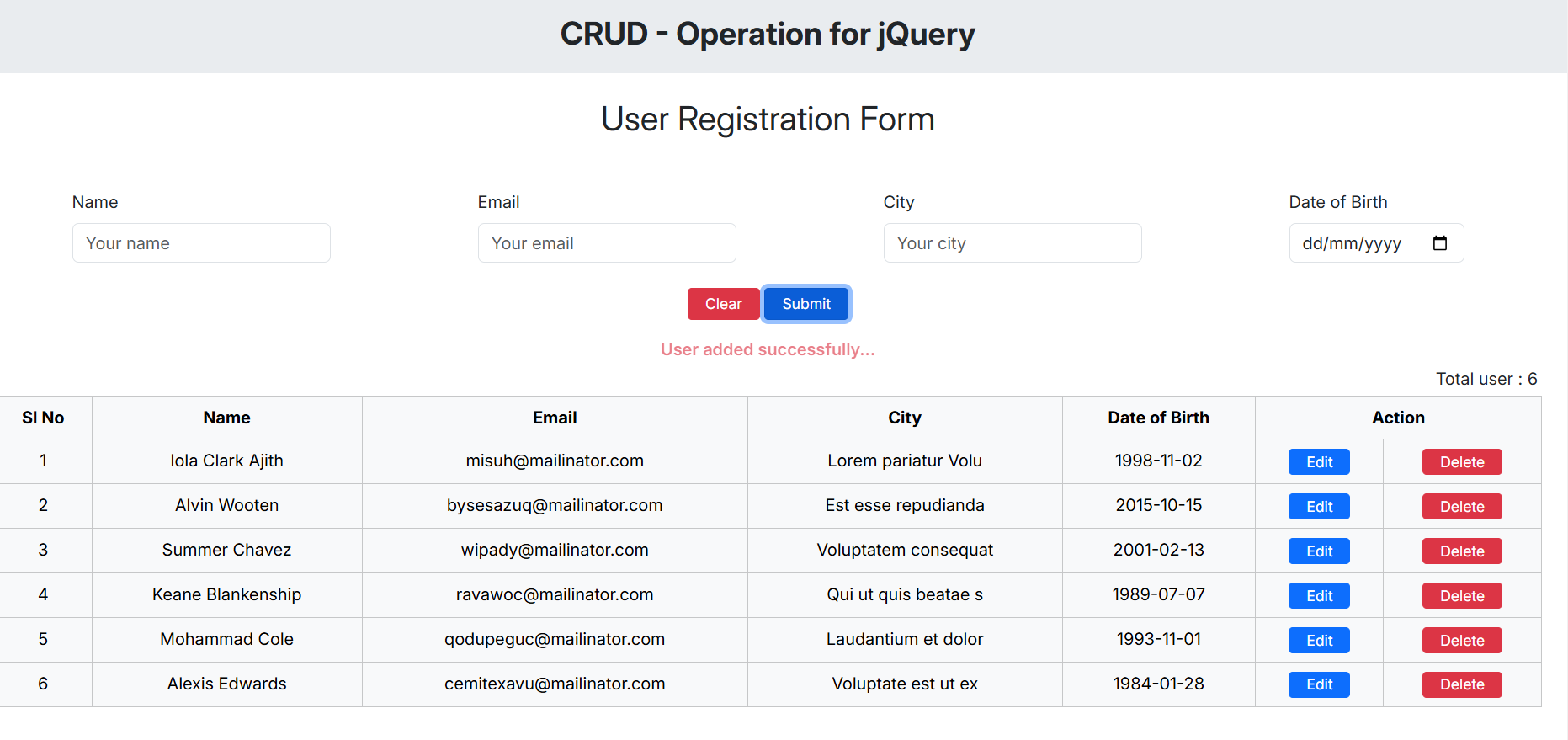
User Registration Form with CRUD Operations Using jQuery
User Registration Form with CRUD Operations Using jQuery
Creating a User Registration Form with CRUD (Create, Read, Update, Delete) operations using jQuery involves several key steps. Here's a detailed explanation and implementation using HTML, jQuery, and JavaScript. This example is designed to manage users by allowing them to register, view a list of users, update their details, and delete them.
Step-by-Step Explanation of CRUD Operations
1. HTML Form (for Create and Update)
The form is used to input the user’s information. It will have fields like Name, Email, and Age. The form allows users to enter data to create a new user or update an existing user's details.
- Input Fields: Name, Email, and Age.
- Submit Button: The button submits the form data.
- Hidden ID: A hidden input field tracks the user ID for updates.
<form id="userForm"> <input type="hidden" id="userId" value=""> <label for="name">Name:</label> <input type="text" id="name" required> <label for="email">Email:</label> <input type="email" id="email" required> <label for="age">Age:</label> <input type="number" id="age" required> <button type="submit">Register User</button> </form>
2. Display User List (for Read)
A table will display all users with options to update or delete their details. This allows the user to see a list of all registered users and perform actions on them.
- Table: Displays user information (Name, Email, Age).
- Action Buttons: Edit and Delete buttons allow interaction with individual users.
<table id="userTable"> <thead> <tr> <th>Name</th> <th>Email</th> <th>Age</th> <th>Actions</th> </tr> </thead> <tbody> <!-- User rows will be dynamically added here --> </tbody> </table>
3. CRUD Operations Using jQuery
This step will involve implementing CRUD operations using jQuery. The data is stored in the browser's memory (in an array).
- Create: Add new users to the list.
- Read: Display the list of users.
- Update: Modify existing user details.
- Delete: Remove a user from the list.
Create: Add New Users
The user can add a new user by filling out the form and submitting it. The data is added to an array and displayed in the table.
Read: Display List of Users
The user list is displayed in a table. Each user is shown with their name, email, and age, along with the option to edit or delete them.
Update: Modify Existing User Details
When the user clicks "Edit", their details are populated in the form, allowing them to update the information. Once the form is submitted again, the user is updated in the array.
Delete: Remove a User
The user can delete a user by clicking the "Delete" button. A confirmation prompt is shown before the user is removed from the list.
Explanation of User Management System
1. HTML Structure:
The form (
#userForm
) contains fields for the name, email, and age of the user. A hidden input (#userId
) is used to track the user ID for updating an existing user.The table (
#userTable
) is used to display the registered users. Each row has two buttons: Edit (to update the user) and Delete (to remove the user).2. CSS Styling:
Simple styles are applied to format the form and table for better readability.
3. jQuery Functions:
- renderUsers(): This function is used to update the user table every time a new user is added, updated, or deleted. It clears the table and then adds all the users from the users array.
- submit(): The form submission is intercepted to handle adding or updating a user. If
userId
is set, the user is updated; otherwise, a new user is added to the list. - editUser(): This function is called when the Edit button is clicked. It populates the form with the user's data and switches the button text to "Update User".
- deleteUser(): This function removes the user from the users array and re-renders the table. It asks for confirmation before deletion.
4. No Backend:
This example doesn't interact with a backend database; instead, it uses an in-memory array (users
) to store the user data. When the page is refreshed, the data is lost.
Key Features:
- Create: Register a new user.
- Read: Display all registered users in a table.
- Update: Edit a user's information.
- Delete: Remove a user from the list.
Usage:
Fill out the registration form and click "Register User" to add a new user.
Edit or delete a user from the user list by clicking the respective buttons.


30+
Project Complete